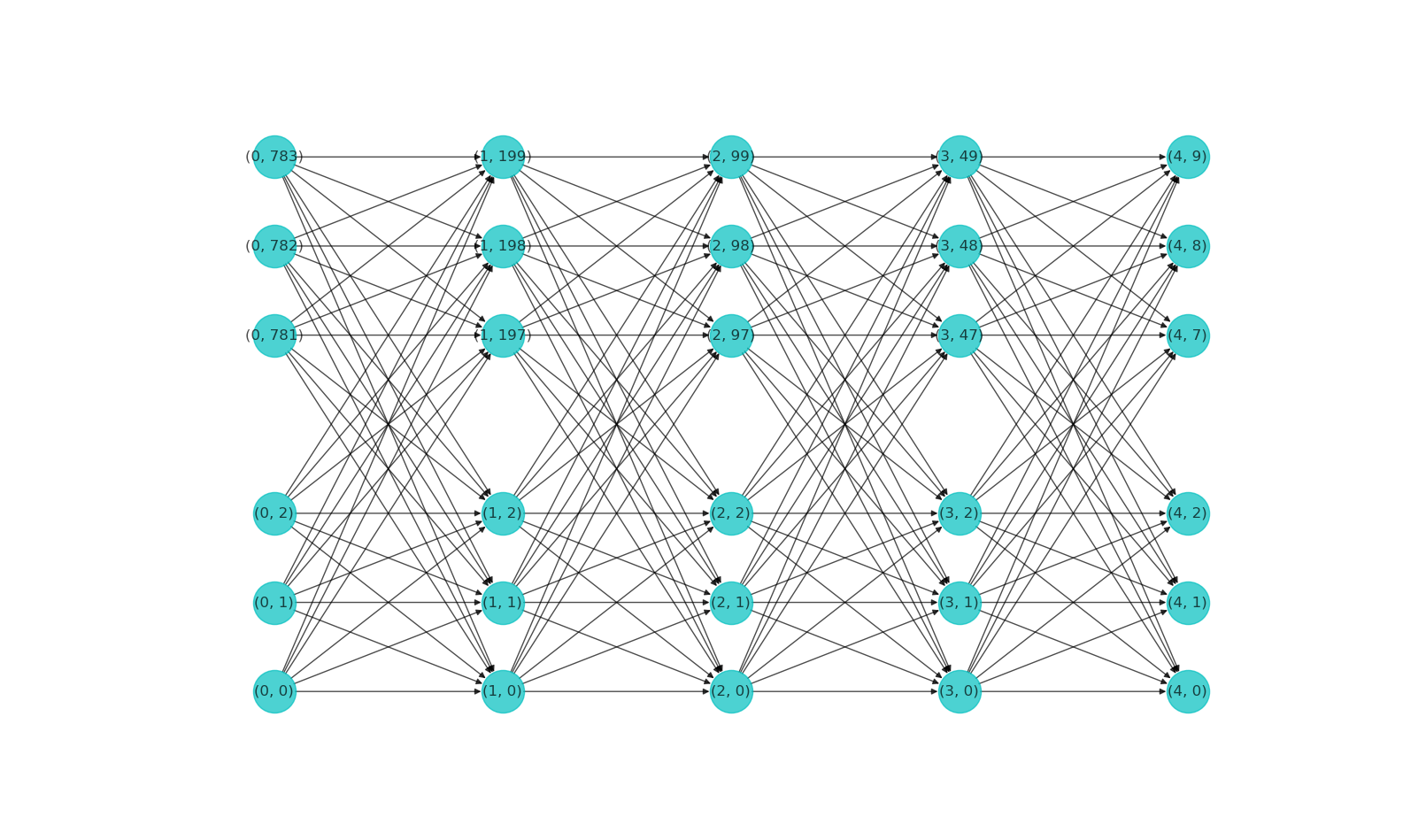
Create a simple neural network using numpy NumberSmithy
B efore we start programming, let's stop for a moment and prepare a basic roadmap. Our goal is to create a program capable of creating a densely connected neural network with the specified architecture (number and size of layers and appropriate activation function). An example of such a network is presented in Figure 1.
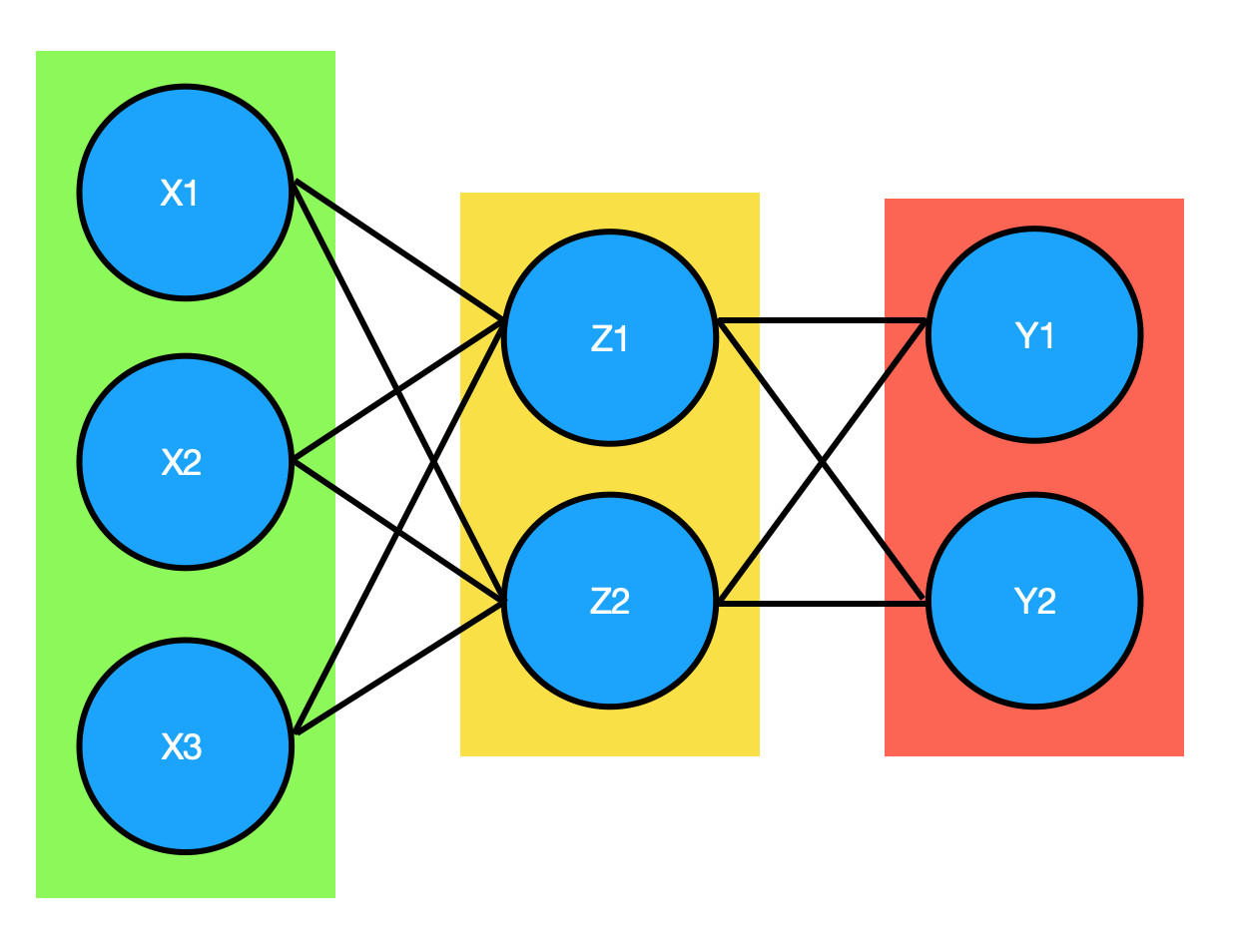
Neural Networks from scratch in python
Neural Network is a collection of neurons (computing units), put in the structure of layers and modeled in the same way the human brain makes it computation. This configuration allows performing.
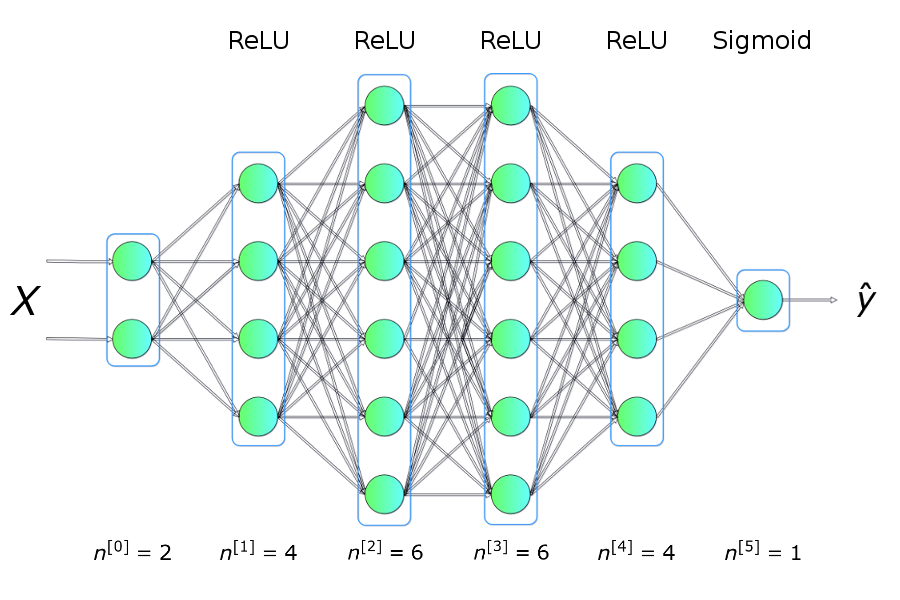
Let’s code a Neural Network in plain NumPy Towards Data Science
NumPyANN is a Python project for building artificial neural networks using NumPy. NumPyANN is part of PyGAD which is an open-source Python 3 library for implementing the genetic algorithm and optimizing machine learning algorithms. Both regression and classification neural networks are supported starting from PyGAD 2.7.0.

Neural Networks in Python A Complete Reference for Beginners AskPython
To associate your repository with the numpy-neural-network topic, visit your repo's landing page and select "manage topics." GitHub is where people build software. More than 100 million people use GitHub to discover, fork, and contribute to over 420 million projects.
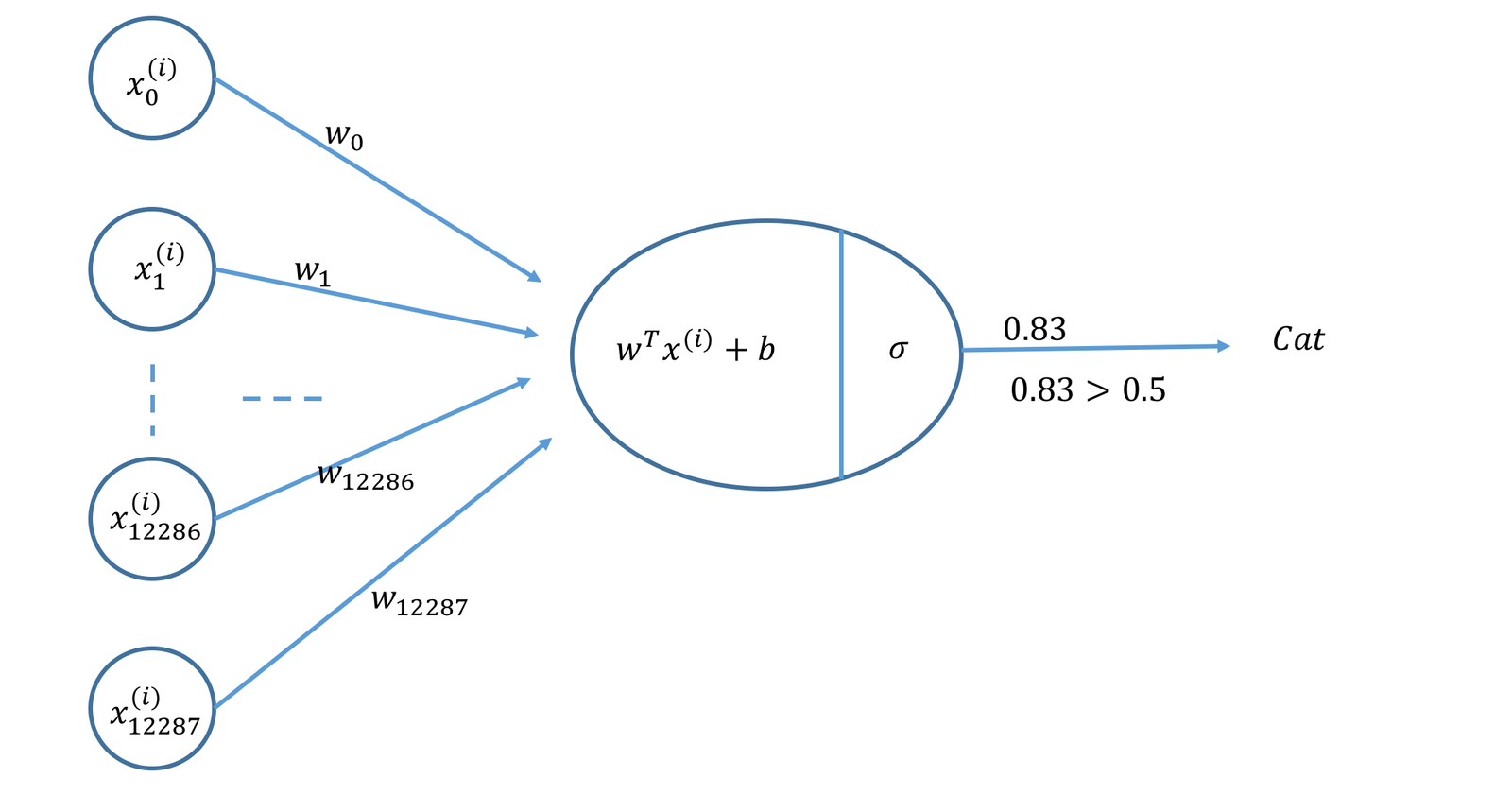
Build a Simple Neural Network using Numpy
Overview. In this article I'll be implementing a deep neural network with 2 hidden layers that uses stochastic descent with momentum and use the MNIST dataset to train and test the model. This dataset is the most commonly used for introductory level deep learning and consists of labelled hand-written digits from 0-9.

Building a Neural Network Only Using NumPy LaptrinhX
First we initialize the weights of the network. So to recap, we need two matrices, that contain the learnable weights (parameters) of the network. Wij with shape (4,10) and Wjk with shape (10,3.

numpy implement Neural Network in python Stack Overflow
Neural Network Regression Implementation and Visualization in Python Neural network regression is a machine learning technique used for solving regression problems. In regression tasks, the goal.
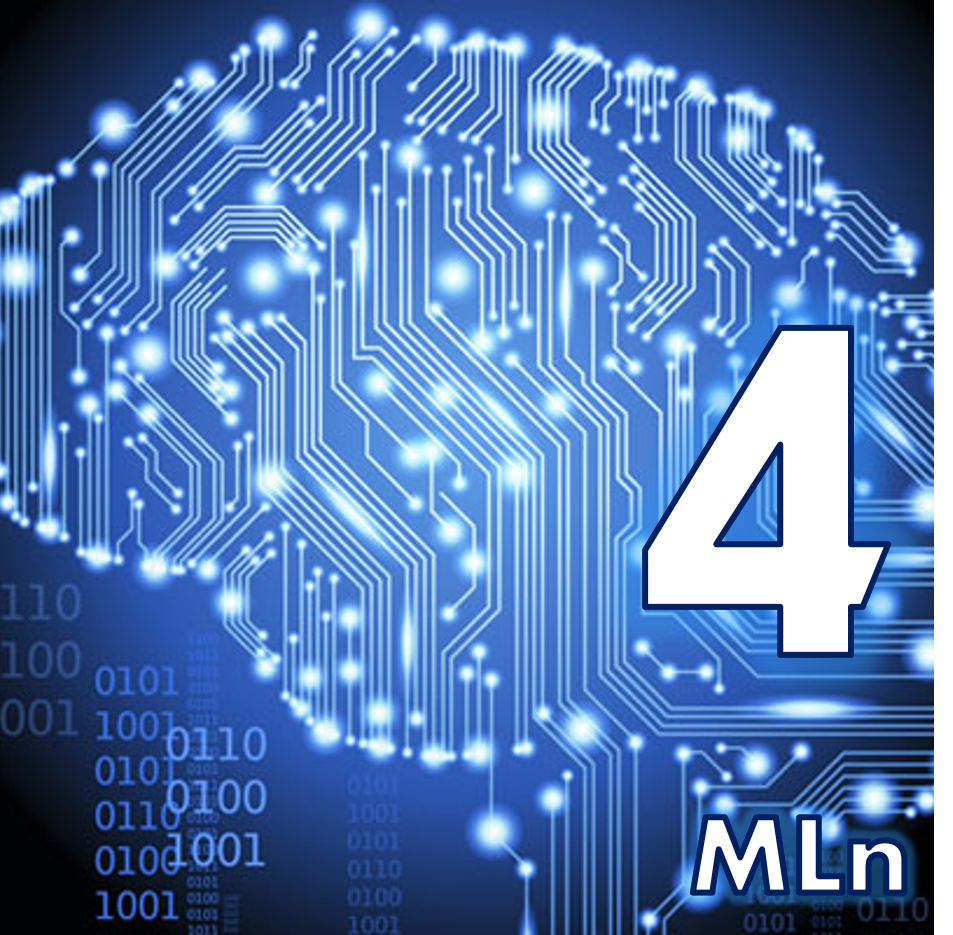
A Simple Neural Network With Numpy in Python · Machine Learning Notebook
Introduction. In this article, I will walk through the development of an artificial neural network from scratch using NumPy. The architecture of this model is the most basic of all ANNs — a simple feed-forward network. I will also show the Keras equivalent of this model, as I tried to make my implementation 'Keras-esque'.

GitHub Simple implementation of MLP neural network in NumPy
"main.py": it's a Python script from where we will run the neural network "utils.py": it's a Python file in which we define the function needed to build the neural network; We will mainly focus on the "utils.py" file since it's where most of the network implementation is. The first function is init_params. It takes as input.

Deep Learning with Python Neural Networks tutorial) (2022)
For the given picture datasets, it can be done by dividing every row of the dataset by 255 (the maximum value of a pixel channel). train_x = train_x/255. test_x = test_x/255. Now we will build a simple neural network model that can correctly classify pictures as cat or non-cat. 3.
Neural Networks with Numpy for Absolute Beginners Introduction
Densely connected neural network. Luckily, the implementation of such a layer is very easy. The forward pass boils down to multiplying the input matrix by the weights and adding bias — a single line of NumPy code. Each value of the weights matrix represents one arrow between neurons of the network visible in Figure 10.

Python Basics with Numpy John Canessa
SGD is a optimizer used for fit the neural network, this technique is based by Gradient Descent. In SGD is used the matriz representation, the equation for represent the update the weights is bellow. Vk+1 =Vk − η. ∇L(Wij) V k + 1 = V k − η. ∇ L ( W i j) W = Vk+1 W = V k + 1.

Numpy Gradient Descent Optimizer of Neural Networks Python Pool
That's because you are using a wrong activation function (i.e. sigmoid). The main reason why we use sigmoid function is because it exists between (0 to 1).Therefore, it is especially used for models where we have to predict the probability as an output.Since probability of anything exists only between the range of 0 and 1, sigmoid is the right choice.

Implement a Neural Network from Scratch with NumPy by Dorian Lazar Towards AI
Step 2: Import Numpy library and Counter function. We'll use a function called counter in our project we'll get to this later, but first let's import it.
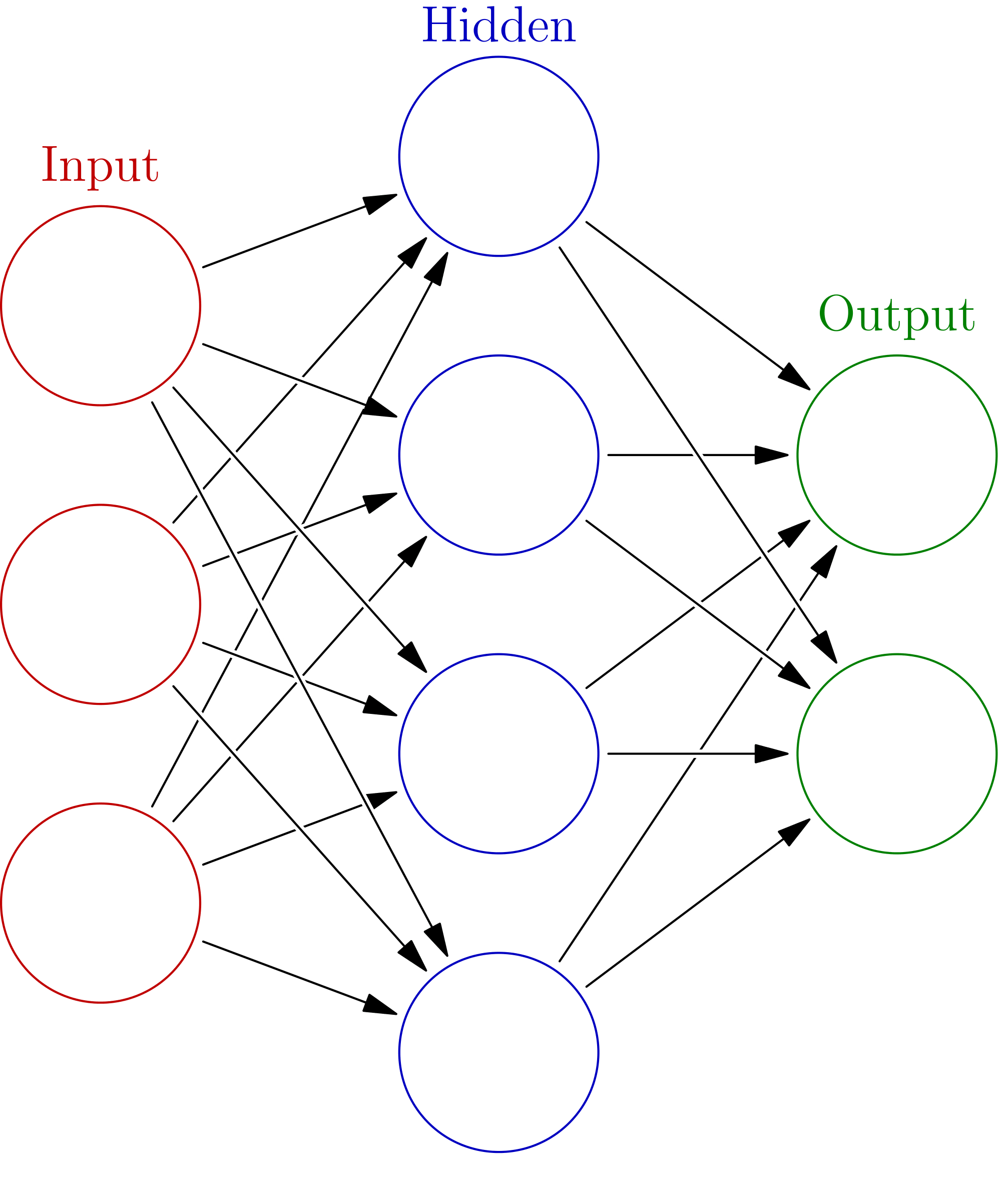
A Beginner’s Guide to Neural Networks in Python and SciKit Learn 0.18 (Jose Portilla) Vichara
In this post we'll build a two-layer neural network from scratch in Python using only the Numpy library. The full code implementation as well as the test example and plots are contained in this Jupyter notebook.. In Python we don't actually need to do this because Numpy will automatically broadcast and add the vector $\mathbf{b}_1$ to.
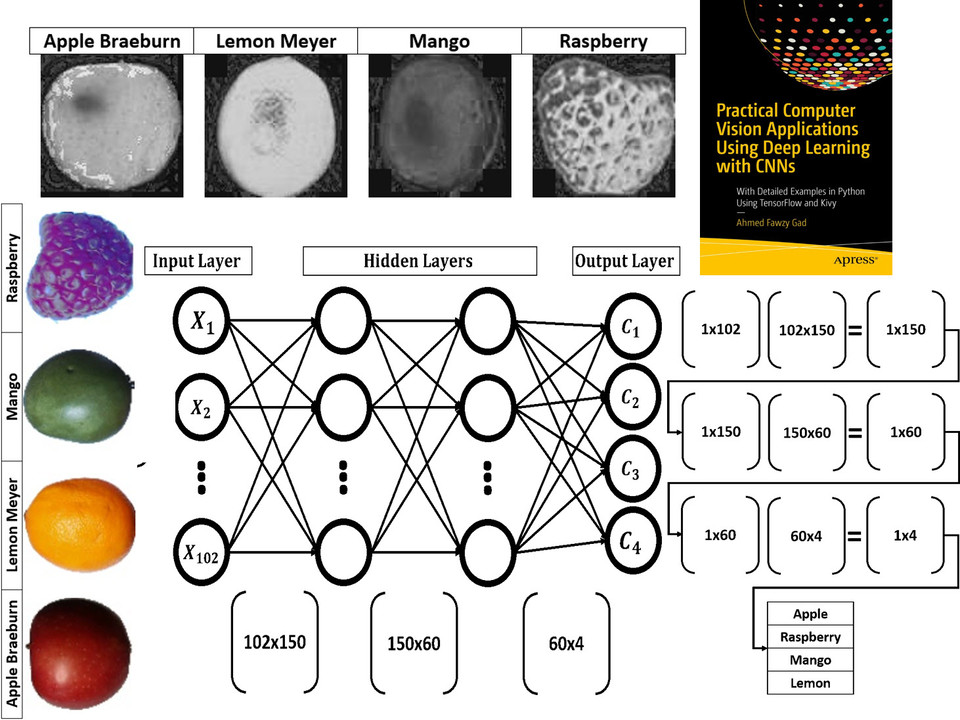
GitHub ahmedfgad/NumPyCNN Building Convolutional Neural Networks From Scratch using NumPy
This means we need to keep a track of the index of the layer we're currently working on ( J J) and the index of the delta layer ( K K) - not forgetting about the zero-indexing in Python: for index in range (self.numLayers): delta_index = self.numLayers - 1 - index. Let's first get the outputs from each layer: